概要
これまで比較的 WinForms を利用したサンプルを掲載してきましたが、WPFでも問題はありません。
.dll 形式である必要もなく、.exeのままでも hm.NET.dll から利用することが出来ます。
WPFのアプリケーションを「.NET Framework」で作成
.NET5/.NET6 といったCore系列ではなく、.NET Frameworkを選択する必要性があるので注意してください。
hm.NET.dllは .NET Frameworkのみの対応です。
.NET5や.NET6などのWPFで制作したい場合は、hm_Hm.NetCOMを利用してください。
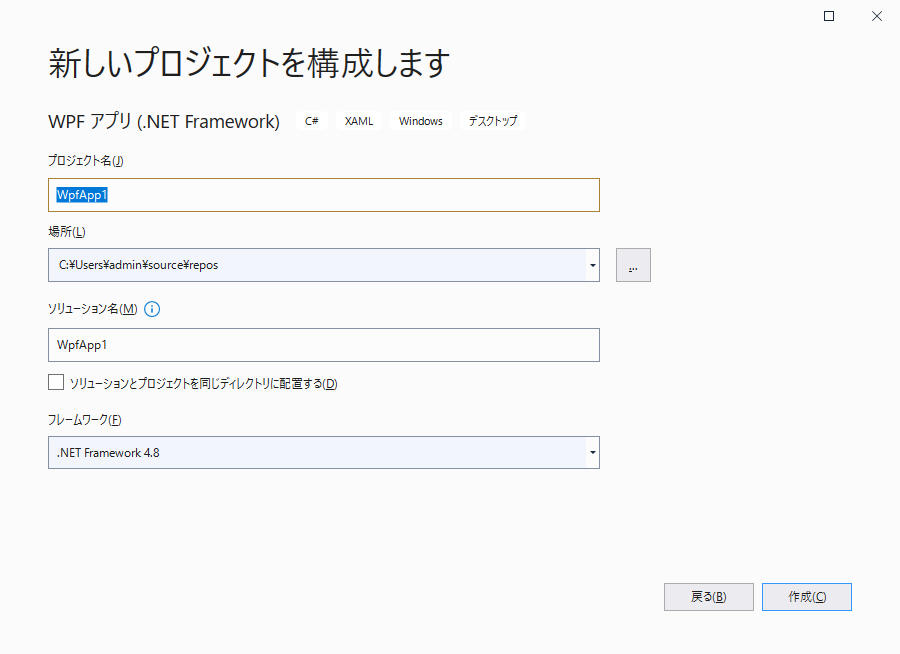
my_button の Click のイベントハンドラからメソッドを自動生成して...
自動生成しなくとも、ご自分で MainWindow.xaml.cs を直接編集する形でももちろんOKです。
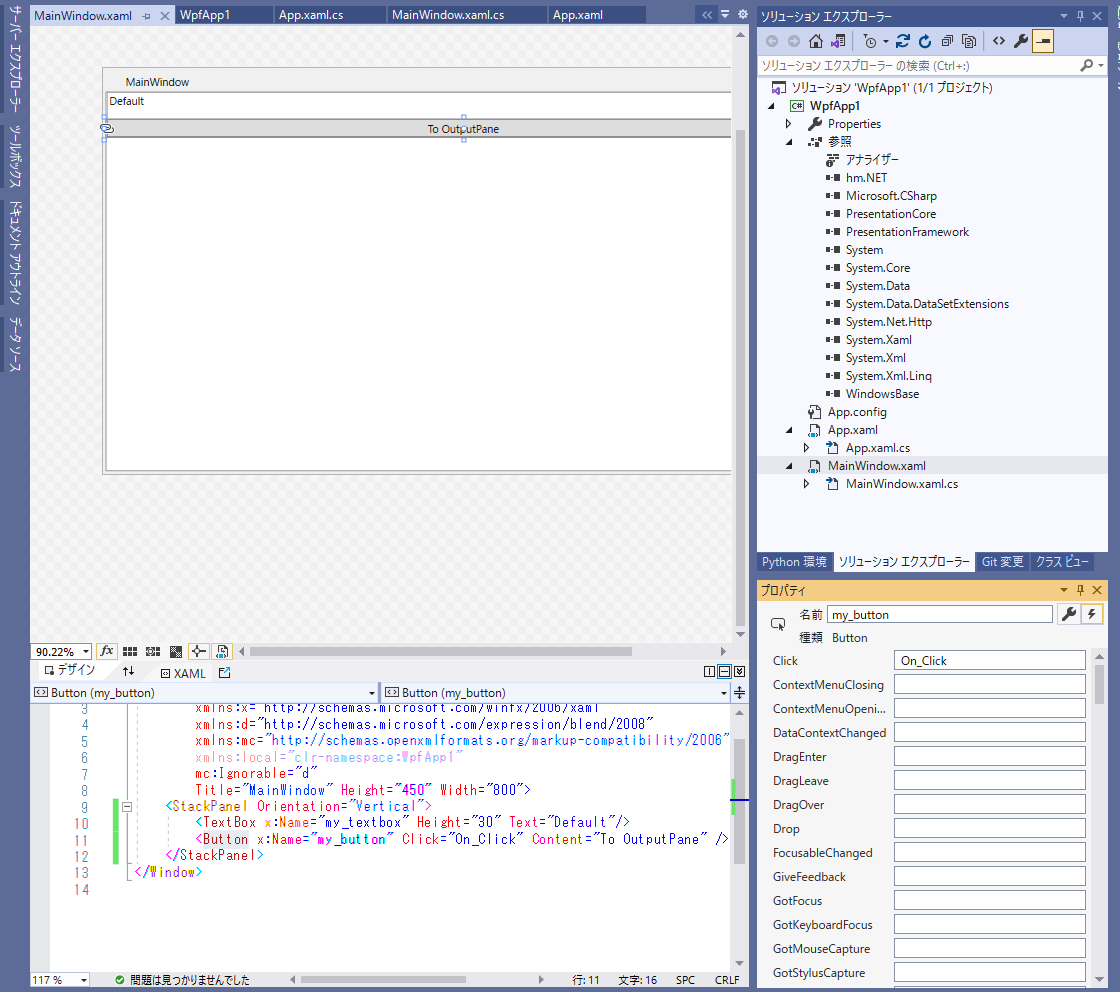
my_textbox の内容を、秀丸のアウトプット枠へと出力する短いソース例にしてみましょう。
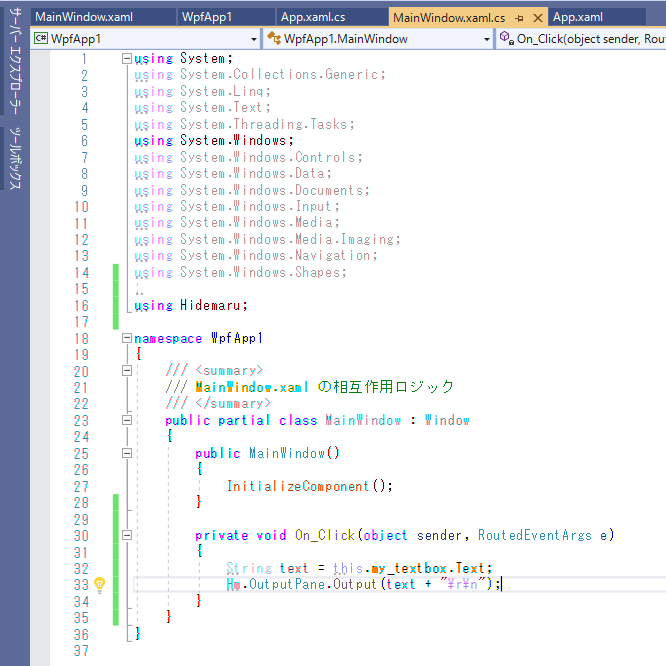
秀丸マクロから直接呼び出されるメソッドを定義
定義場所に迷うところですがが、 App.xaml.cs が空っぽですので、ここが良いでしょう。
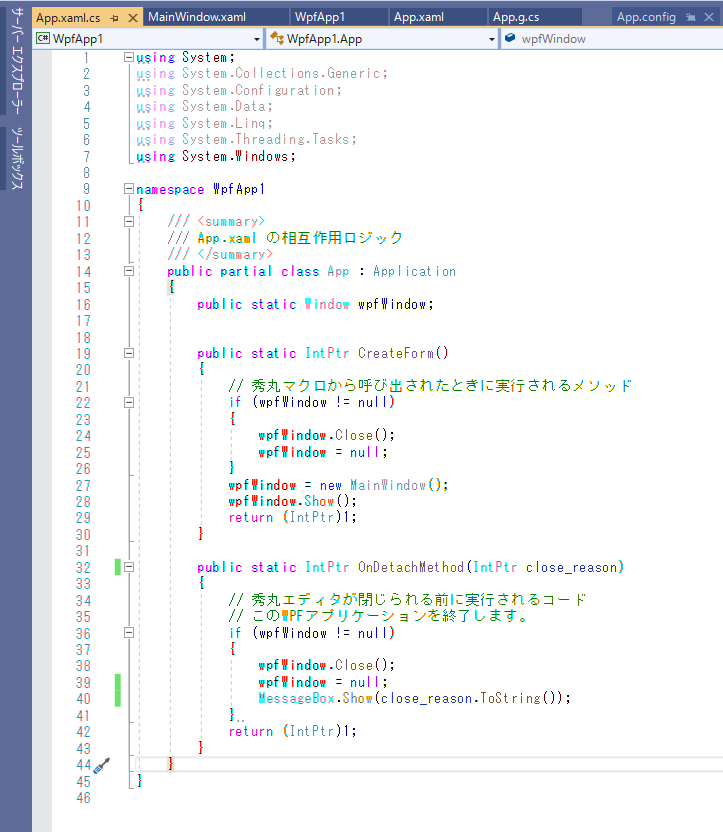
秀丸マクロ側のソース
呼び出し側のマクロを用意しましょう。
WpfApp1.exeと、このWpfWpfTest.mac の2つのファイルを同一のディレクトリに配置して、マクロを実行してみましょう。
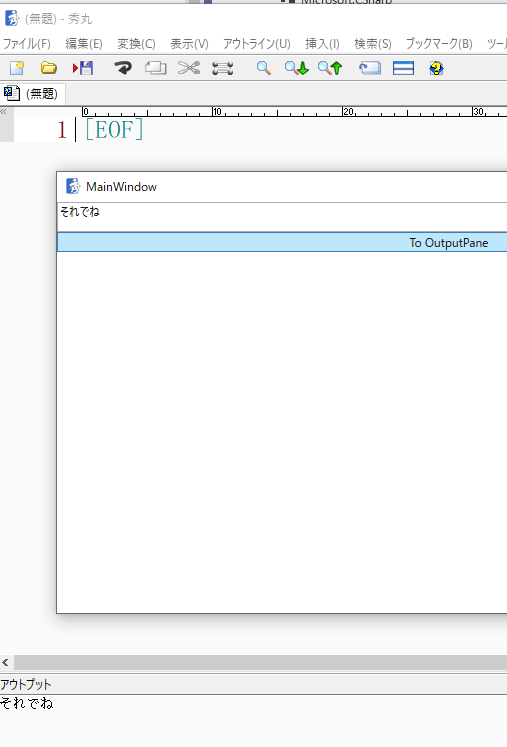
On_Click は非同期メソッドなので...
非同期メソッド中は、通常マクロ中ではないため、hm.NET.dllで利用できる機能が制限されてしまいます、
それを打開する Hm.Macro.Exec.DoMethod(...) があることを思い出してください。
MainWindowのインスタンス(this)を static メソッドから参照するため、static変数 self へと this を伝搬しているところが工夫となります。
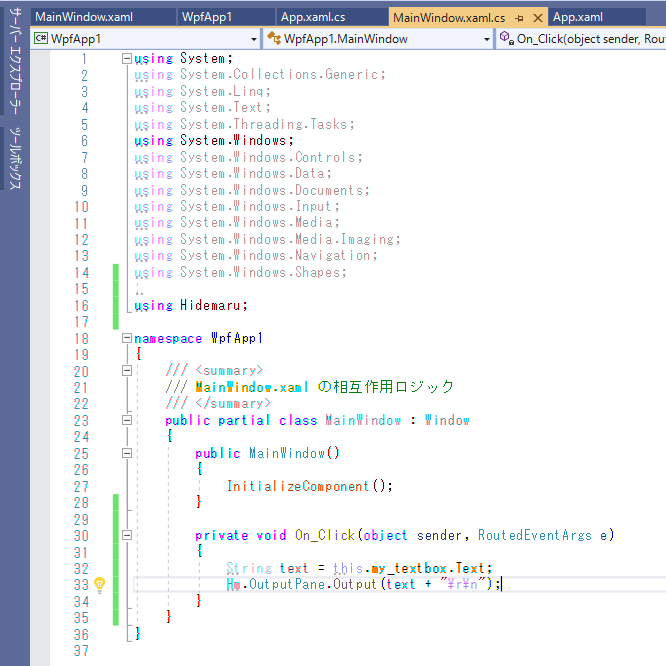
Hm.Macro.Exec.DoMethod を利用することで、非同期メソッド中であっても、秀丸マクロを「改めて実行」するため、
Hm.Macro.Var["..."] や Hm.Macro.Funtion(...)、Hm.Macro.Statement(...) が利用可能となります。
これにより、非同期中独特の制限が大きく解けて、秀丸側から提供されているマクロ関数等の機能を利用しやすくなります。